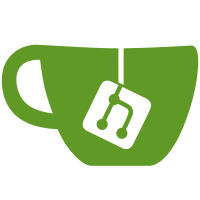
This commit fixes issue #91. [AN10922][] specifies the key diversification algorithms used by the MIFARE SAM AV3. Support for these algorithms was added to `libfreefare` via pull-request #79. However, while every attempt was made to write a faithful implementation, the implemented code did not properly handle cases where the diversification data was less than or equal to the block size of the cipher: 16 bytes for AES, and 8 bytes for DES. This bug was identified in issue #91. This commit addresses this problem while providing a way to revert to the previous behavior in cases where it is necessary to maintain previous deployments. This was accomplished by introducing a new `flags` parameter to the `mifare_key_deriver_new_an10922` method. Normally, `flags` should simply be set to `AN10922_FLAG_DEFAULT`. However, if the previous behavior is required, it should be set to `AN10922_FLAG_EMULATE_ISSUE_91`. [AN10922][] does not include any test vectors that might have helped to identify this problem earlier. However, [AN10957][] (pages 13-14) was found to have a suitable example usage of [AN10922][] with an appropriately short value for *M* that we are using as a test vector to verify correct behavior. Note that the issue being addressed here is not a security issue: using the `AN10922_FLAG_EMULATE_ISSUE_91` should not be any less secure than using `AN10922_FLAG_DEFAULT`. [AN10922]: https://www.nxp.com/docs/en/application-note/AN10922.pdf [AN10957]: https://www.nxp.com/docs/en/application-note/AN10957.pdf
96 lines
2.7 KiB
C
96 lines
2.7 KiB
C
#include <err.h>
|
|
#include <stdlib.h>
|
|
|
|
#include <nfc/nfc.h>
|
|
|
|
#include <freefare.h>
|
|
|
|
int
|
|
main(int argc, char *argv[])
|
|
{
|
|
int error = EXIT_SUCCESS;
|
|
nfc_device *device = NULL;
|
|
FreefareTag *tags = NULL;
|
|
|
|
if (argc > 1)
|
|
errx(EXIT_FAILURE, "usage: %s", argv[0]);
|
|
|
|
nfc_connstring devices[8];
|
|
size_t device_count;
|
|
|
|
nfc_context *context;
|
|
nfc_init(&context);
|
|
if (context == NULL)
|
|
errx(EXIT_FAILURE, "Unable to init libnfc (malloc)");
|
|
|
|
device_count = nfc_list_devices(context, devices, sizeof(devices) / sizeof(*devices));
|
|
if (device_count <= 0)
|
|
errx(EXIT_FAILURE, "No NFC device found");
|
|
|
|
for (size_t d = 0; d < device_count; d++) {
|
|
if (!(device = nfc_open(context, devices[d]))) {
|
|
warnx("nfc_open() failed.");
|
|
error = EXIT_FAILURE;
|
|
continue;
|
|
}
|
|
|
|
if (!(tags = freefare_get_tags(device))) {
|
|
nfc_close(device);
|
|
errx(EXIT_FAILURE, "Error listing tags.");
|
|
}
|
|
|
|
for (int i = 0; (!error) && tags[i]; i++) {
|
|
switch (freefare_get_tag_type(tags[i])) {
|
|
case MIFARE_ULTRALIGHT:
|
|
case MIFARE_ULTRALIGHT_C:
|
|
break;
|
|
default:
|
|
continue;
|
|
}
|
|
|
|
char *tag_uid = freefare_get_tag_uid(tags[i]);
|
|
printf("Tag with UID %s is a %s\n", tag_uid, freefare_get_tag_friendly_name(tags[i]));
|
|
if (freefare_get_tag_type(tags[i]) == MIFARE_ULTRALIGHT_C) {
|
|
FreefareTag tag = tags[i];
|
|
int res;
|
|
MifareDESFireKey key;
|
|
uint8_t key1_3des_data[16] = { 0x49, 0x45, 0x4D, 0x4B, 0x41, 0x45, 0x52, 0x42, 0x21, 0x4E, 0x41, 0x43, 0x55, 0x4F, 0x59, 0x46 };
|
|
key = mifare_desfire_3des_key_new(key1_3des_data);
|
|
if (mifare_ultralight_connect(tag) < 0)
|
|
errx(EXIT_FAILURE, "Error connecting to tag.");
|
|
res = mifare_ultralightc_authenticate(tag, key);
|
|
if (res != 0) {
|
|
MifareDESFireKey diversified_key = NULL;
|
|
MifareKeyDeriver deriver = mifare_key_deriver_new_an10922(key, MIFARE_KEY_2K3DES, AN10922_FLAG_DEFAULT);
|
|
|
|
mifare_key_deriver_begin(deriver);
|
|
mifare_key_deriver_update_uid(deriver, tag);
|
|
diversified_key = mifare_key_deriver_end(deriver);
|
|
|
|
// Disconnect and reconnect.
|
|
mifare_ultralight_disconnect(tag);
|
|
if (mifare_ultralight_connect(tag) < 0)
|
|
errx(EXIT_FAILURE, "Error connecting to tag.");
|
|
|
|
res = mifare_ultralightc_authenticate(tag, diversified_key);
|
|
|
|
printf("Authentication with default key: %s\n", res ? "fail" : "success (diversified)");
|
|
|
|
mifare_desfire_key_free(diversified_key);
|
|
mifare_key_deriver_free(deriver);
|
|
} else {
|
|
printf("Authentication with default key: success\n");
|
|
}
|
|
mifare_desfire_key_free(key);
|
|
mifare_ultralight_disconnect(tag);
|
|
}
|
|
free(tag_uid);
|
|
}
|
|
|
|
freefare_free_tags(tags);
|
|
nfc_close(device);
|
|
}
|
|
|
|
nfc_exit(context);
|
|
exit(error);
|
|
}
|